- The differences in tuple, dictionary, set and list
- List Comprehension
- Using slicing in matrix selection
The differences in tuple, dictionary, set and list
tuple : immutable , i.e. user cannot change the content of the tuple
dictionary : accessible by key-value pair
list : list of items
set : non repeatable list
Code Sample
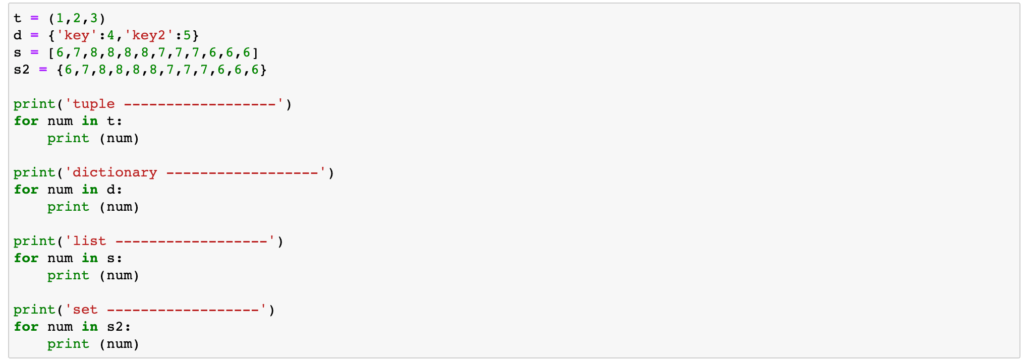
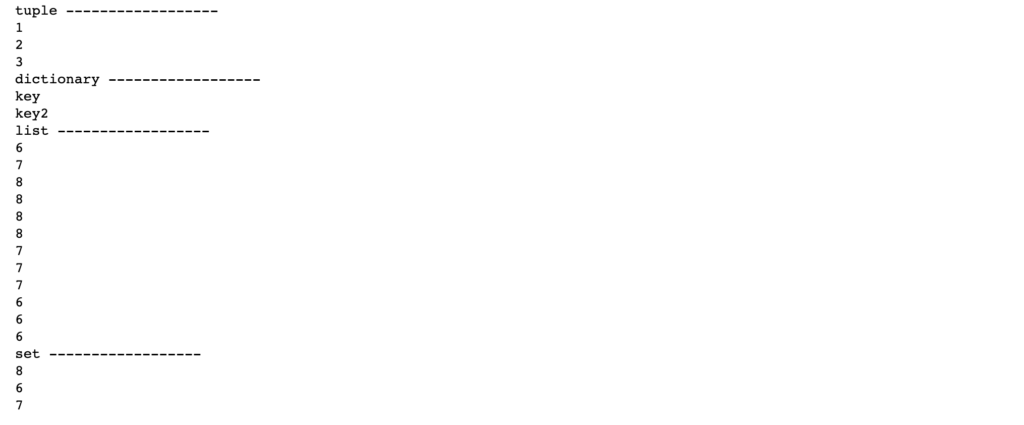
List comprehension
Give a list of items in python, it is common to want to perform similar tasks on the items. The common way to perform the task would be to write a for loop to iterate over each item in the list.

In the above example, we composed a for loop that iterate over each item in the list x and take square value of each item in the list.
In list comprehension, we have shorthand for the above task. Essentially code that perform the same tasks but with less coding.

What else can we do with list comprehension ?
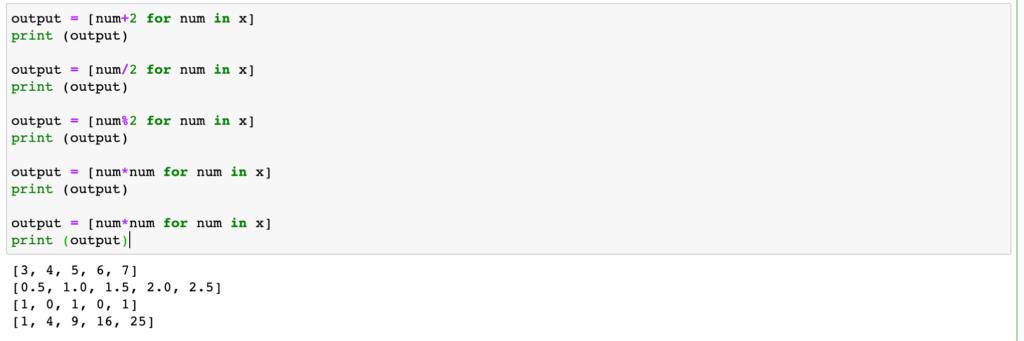
Personally I think that list comprehension etc makes the code harder to comprehend for newbie. There is a good article that provides better overview of the topic.
Using slicing in matrix selection
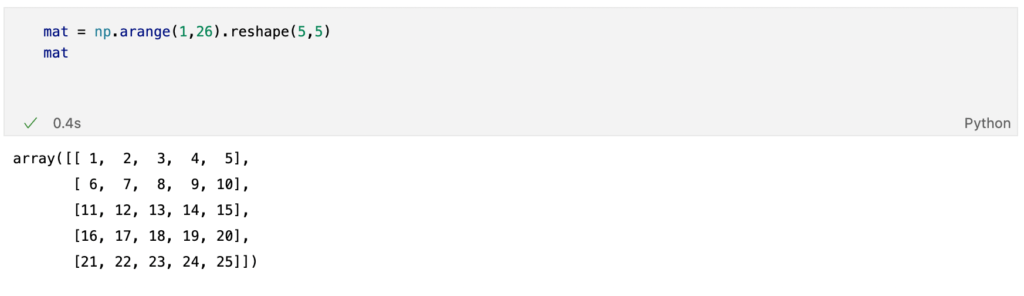
Let’s say we create a matrix of 25.
Using mat [:,:], we will select all from first row and all from first column.
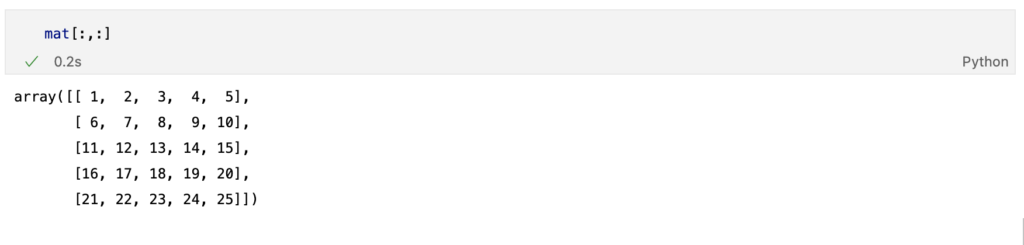
Using mat[:,0], we are selecting all from first row and that of first column. Note that the orientation is changed.

To get it in a different orientation, use the mat[ :,0:1 ] notation.
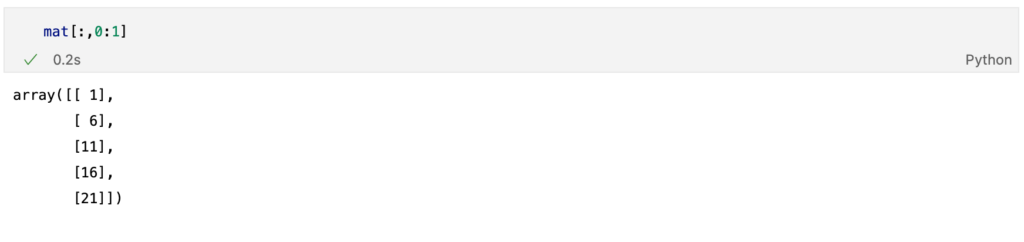
To return 3 elements in each row, use mat[ :,0:3] notation.
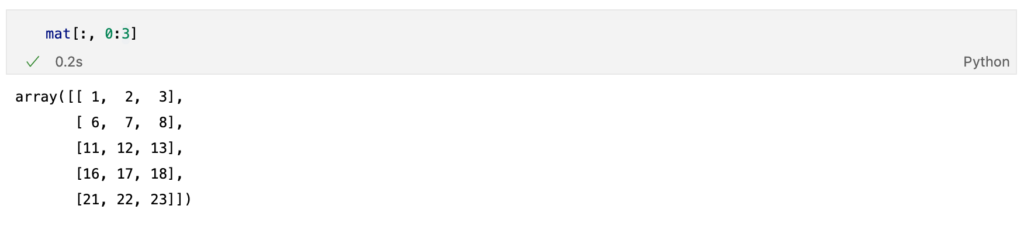
To return 2×2 elements back, we can than use mat[ 0:2 , 0:2 ]

Essentially, using the selection notation always return a 1 x n matrix. The : notation will determine the entries.